Table Of Content
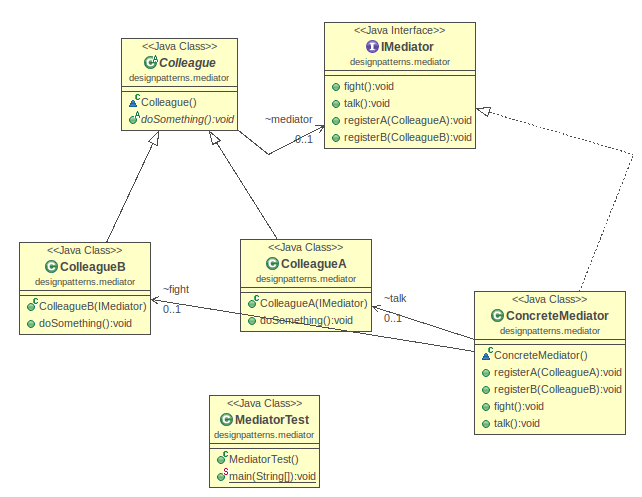
The Mediator Pattern, which is similar to the Command, Chain of Responsibility, and Iterator patterns, are part of the Behavioral pattern family of the Gang of Four design patterns. Behavioral patterns address responsibilities of objects in an application and how they communicate between them. The Mediator pattern allows the loose coupling between set of objects in an application by handling the interactions between the objects. Note that the mediator design pattern differs from the facade design pattern. The mediator pattern facilitates how a set of objects interact, while the facade pattern simply provides a unified interface to a set of interfaces in the application.
Source Code Implementation
However, the benefits of an application composed of a large number of small objects comes with a challenge – the communication between them. Objects need to communicate to perform business requirements, and as the number of objects grow, connections between the objects required can quickly become unmanageable. In addition objects communicating between them needs to know about each other – they are all tightly coupled that violates the basics of the SOLID principles. The Mediator pattern is a proven solution to address such recurring challenges and the problems arising out of them. This will be a class implementing the communication operations of the Mediator Interface. So, Create a class file named ConcreteFacebookGroupMediator.cs and then copy and paste the following code.
C# Mediator Design Pattern
This pattern helps reduce (or eliminate) coupling between modules in the system by moving that coupling into a Mediator module or class. The Mediator is responsible for coordinating and controlling the interactions of the various modules. This structural code demonstrates the Mediator pattern facilitating loosely coupled communication between different objects and object types.
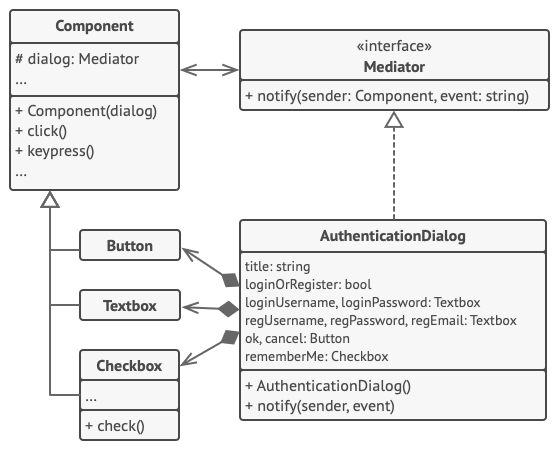
The Mediator Pattern in the Spring Framework
When you wish to reuse component classes in multiple situations, this interface is critical. You can link the component with a different mediator implementation as long as the component communicates with its mediator using the general interface. The Mediator interface specifies communication methods with components, which typically comprise only a single notification method. The Main method of the Program class is going to be the client.
Ø If the state of any of the members changed, it will inform the mediator to do whatever depends on it. Again, the caller member simply does not care what depends on it. Ø If any member needs anything from the other members, it will just inform the mediator and it will handle the order it by its own way. The caller member does not care how the request will be handled.
Real world example of mediator pattern
From the standpoint of a component, everything appears to be a whole black box. The sender has no idea who will handle the request, and the recipient has no idea who issued the request in the first place. Here, the live captioning system acts as a mediator aiding in the communication process. If they attempt direct communication, they tend to send wrong signals and confuse each other even more. The captioning system will act as a router between the people on the call and has its own logic to give a means of communication.
Design of nanoformulation of specialized pro-resolving mediators to facilitate inflammation resolution and disease ... - ScienceDirect.com
Design of nanoformulation of specialized pro-resolving mediators to facilitate inflammation resolution and disease ....
Posted: Tue, 12 Sep 2023 19:26:57 GMT [source]
Creational Design Patterns
So, please modify the Main method of the Program class as shown below. Here, we create the FacebookGroupMediator object, create several Facebook users, and then register all the users to the mediator object. Then, we send a message to the Mediator using the Dave and Rajesh user. The following client code is self-explained, so please go through the comment lines for a better understanding. These are the classes that communicate with each other via the mediator.
Instead of having the pilots talk to each other directly, which would probably end up being quite chaotic, the pilots talk the air traffic controller. The air traffic controller makes sure that all planes receive the information they need in order to fly safely, without hitting the other airplanes. In no way, the participants will communicate directly with each other.
The communication between the components can get rather confusing if there is a large number of components. The connection is often made in the connector of the component, where a mediator object is supplied as a parameter. Concrete Mediators contain the interactions of distinct components. Concrete mediators frequently maintain track of all the components they govern and, in some cases, manage their whole lifespan. The Mediator is an entity that governs how a group of items communicate with one another.
Mediator promotes loose coupling by keeping objects from referring to each other explicitly, and it lets us vary their interaction independently. Design patterns are used to solve common design problems and reduce the complexities in our code. The mediator pattern is a behavioral design pattern that promotes loose coupling between objects and helps to organize the code for inter-object communications. Mediator is a behavioral design pattern that reduces coupling between components of a program by making them communicate indirectly, through a special mediator object.
MediatorPatternDemo, our demo class, will use User objects to show communication between them. In a smart house, there are four smart workers [Alarm, Coffeepot, Calendar, and Sprinkler]; each one of them can do its specific staff as predefined from the owner. When the time comes, the alarm informs the Coffee Machine to start brewing the coffee. But there are more complex conditions, For example, In weekends, the routine will differ. When something important occurs within or to a component, it must only notify the mediator. If the mediator receives a notification, it can easily identify the sender, which might be just enough to determine which component should be triggered.
If we were to model this in software, we could decide to have Userobjects coupled to Group objects, and Group objects coupled to Userobjects. Then when changes occur, both classes and all theirinstances would be affected. Although we’re hopefully not controlling airplanes in JavaScript, we often have to deal with multidirectional data between objects.
The Mediator Design Pattern reduces the communication complexity between multiple objects. This design pattern provides a mediator object, which will be responsible for handling all the communication complexities between different objects. In the following example, a Mediator object controls the values of several Storage objects, forcing the user code to access the stored values through the mediator.